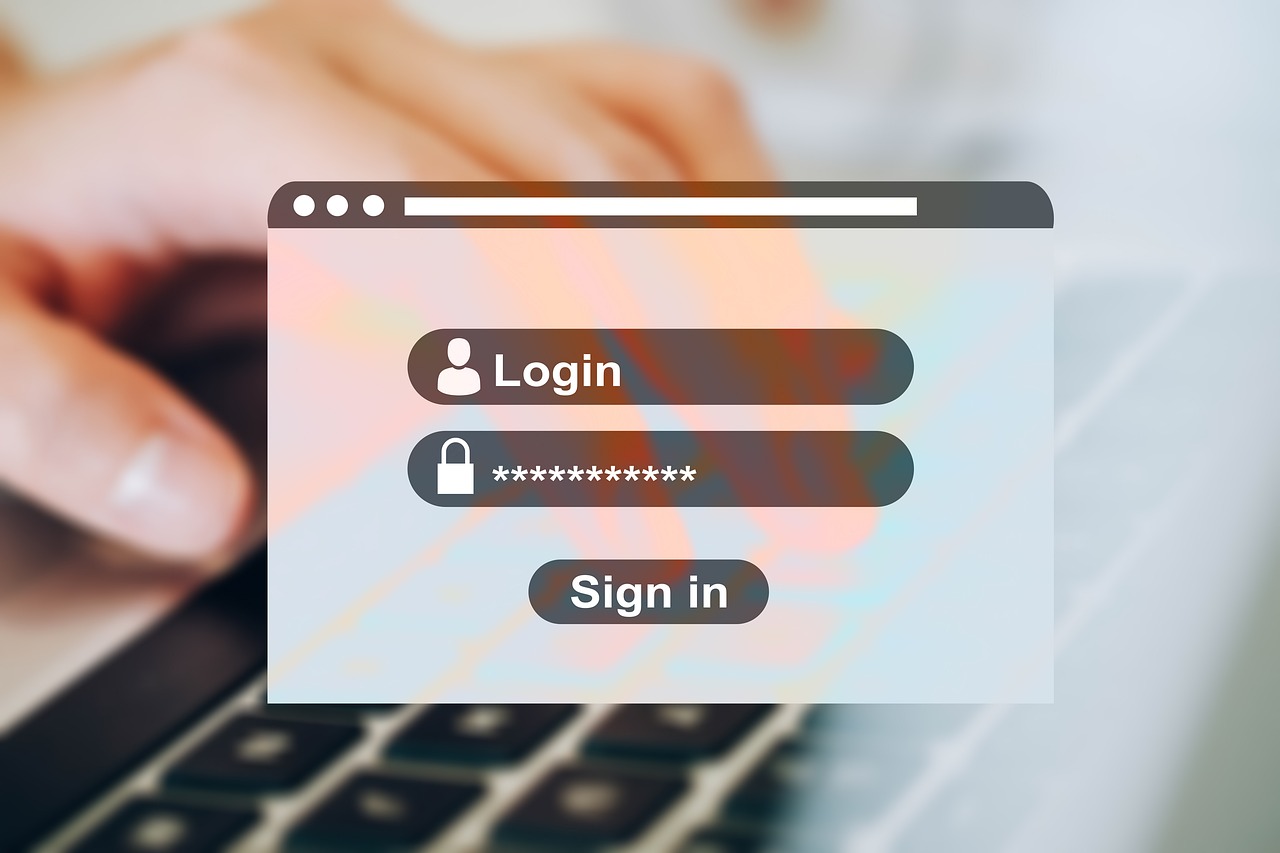
Are you looking for a secure way to manage your team’s secrets in a hybrid working environment? At Process Squad, we’ve implemented Azure Key Vault to manage secrets and avoid hardcoded passwords in our GitHub code. This has significantly enhanced the security of our applications.
Why Azure Key Vault? Azure Key Vault is a cloud-based service provided by Microsoft that allows you to securely store and manage cryptographic keys, certificates, and secrets used by cloud applications and services. Here are its key features:
- Secrets Management: Securely store and control access to tokens, passwords, certificates, API keys, and other secrets.
- Key Management Solution: Easily create and control the encryption keys used to encrypt your data.
- Certificate Management: Effortlessly provision, manage, and deploy public and private TLS/SSL certificates.
- Distribution Control: Centralize the storage of application secrets, reducing the chances of accidental leaks.
The Dangers of Hardcoding Passwords
Hardcoding passwords in your code is a dangerous practice that could compromise system security. It allows all developers on a project to view the password and makes remediation extremely difficult.
At Process Squad, we believe in sharing our learnings with others. Below, we’ve detailed the steps necessary to use Azure Key Vault in Python.
Azure CLI
First, we need to install Azure CLI running the following PowerShell command as administrator on Windows 10/11:
$ProgressPreference = ‘SilentlyContinue’; Invoke-WebRequest -Uri https://aka.ms/installazurecliwindows -OutFile .\AzureCLI.msi; Start-Process msiexec.exe -Wait -ArgumentList ‘/I AzureCLI.msi /quiet’; rm .\AzureCLI.msi
After the installation is completed, you can run the following command to see if it was successful:
az
More information on the installation procedure at Microsoft (https://learn.microsoft.com/en-us/cli/azure/install-azure-cli-windows?tabs=azure-cli).
Azure Login
Run the following PowerShell command as administrator on Windows 10/11 to login to your Azure account:
az login
This will bring you to the default browser to complete the login.
Create Key Vault
Run the following PowerShell command as administrator on Windows 10/11 to create a Resource Group in Azure:
az group create –name “Authentication” –location “WestEurope”
Run the following PowerShell command as administrator on Windows 10/11 to create a Key Vault in Azure:
az keyvault create –name “test-keyvault” –resource-group “Authentication” –location “WestEurope”
You can access it through the VAULT_URL in the code:
https://test-keyvault.vault.azure.net/
Create Key Vault Secret
Run the following PowerShell command as administrator on Windows 10/11 to create an Azure Key Vault Secret (replace vault name with a name of your choice):
az keyvault secret set –vault-name “test-keyvault” –name “ExamplePassword” –value “password”
Run the following PowerShell command as administrator on Windows 10/11 to return the value of an Azure Key Vault Secret:
az keyvault secret show –name “ExamplePassword” –vault-name “test-keyvault” –query “value”
You can also view and manage the secrets on the Azure Portal.
Python
In order to use Azure Key Vault in your python code you need to run the following pip command to get the necessary packages:
pip install –upgrade azure-keyvault-secrets azure-identity azure-mgmt-resource
Here is a sample code to get the secret “ExamplePassword” and its value:
from azure.identity import DefaultAzureCredential
from azure.keyvault.secrets import SecretClient
VAULT_URL = “https://test-keyvault.vault.azure.net/”
credential = DefaultAzureCredential(additionally_allowed_tenants=[‘*’])
client = SecretClient(vault_url=VAULT_URL, credential=credential)
secret = client.get_secret(“ExamplePassword”)
print(f”Secret value is {secret.value}”)
Voila! We hope you find this useful.
#AzureKeyVault #Python #ProcessSquad #Insights